Lists: Inserting and Deleting from Queues
Lists: Inserting and Deleting from Queues
In the real world, a queue isn't always the perfect structure that always lets everyone through in the order they entered. Sometimes you get bored standing around for that roller coaster and decide to ride the oversized teacups one more time. Or maybe you get a fever that can only be cured with more funnel cake.
Hey. It happens.
Or maybe that kid from your English class did their friends a solid and held their place in line while those friends got funnel cake, adding more people to the middle of the line. Or maybe you pick a fight with that kid (because you've been waiting three and a half hours without any funnel cake and you don't think it's fair that the kid gets to both eat funnel cake and make the line longer while you suffer) and it becomes so big that security kicks you out of the park.
Hey. It happens. Especially when funnel cake's on the line.
Point being, the length and composition of a line can change.
Insertions
In computer science, you'll probably need to change your queue all the time, but queues aren't too forgiving about it (probably because you can't bribe them with funnel cake.)
Inserting at the queue's back or removing from the front is easy because queues are meant to work that way. Inserting at the front or removing from the back? Not so much. Even the middle can be a pain to mess with. To do any of these, you'll need an extra queue off to the side to catch elements as they fall.
Let's start with inserting at the front. The original queue (Queue 1) looks like this:
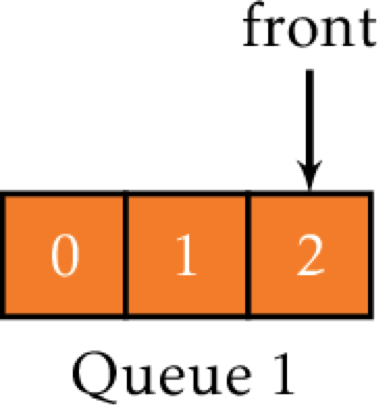
To insert 3 at the front of this queue, you first need to dequeue all of Queue 1 and enqueue each element onto Queue 2. You're essentially copying everything over into a new queue.
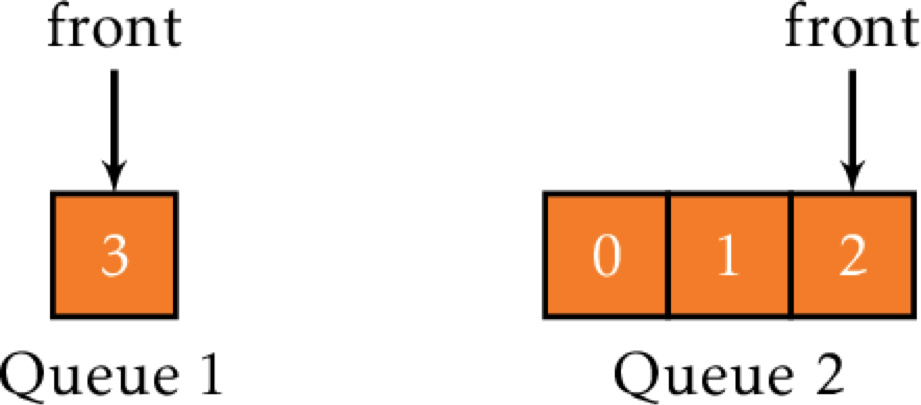
In the empty Queue 1, just enqueue 3. Then you can enqueue the rest of Queue 2 and, because adding elements happens at the back of the queue, everything will stay in order.
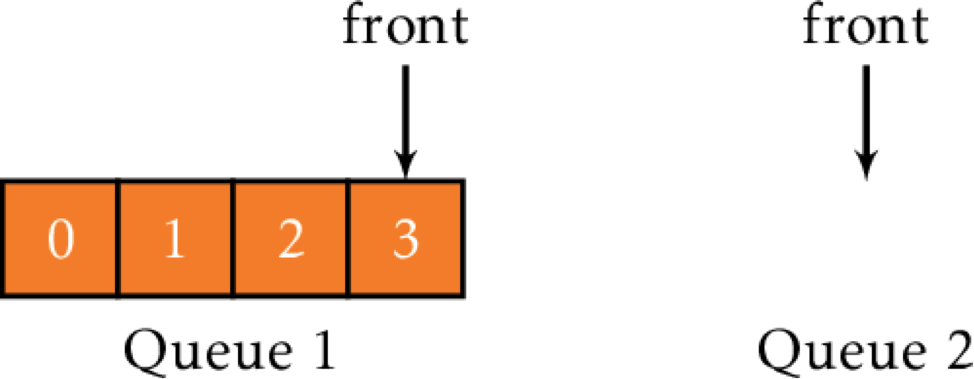
Done.
Deletions
Deleting at the back of a queue takes about as much work—just in reverse this time.
We start with Queue 1, and we want to remove 0.
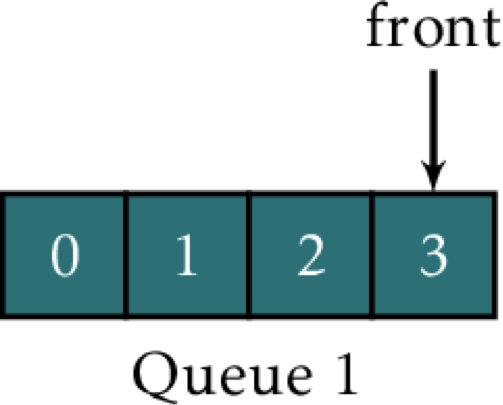
Then all we need to do is dequeue and enqueue everything except 0 onto Queue 2. Those are all the elements we want to save. The only thing left in Queue 1 is—you guessed it—the element we want to delete.
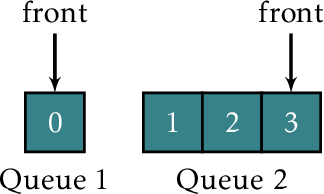
Just dequeue 0 without enqueueing it and you have your 0-less queue.
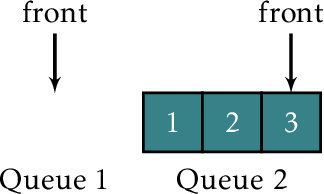
Here comes the fun trick: inserting and deleting elements from the middle of the queue. It'll be fine as long as you remember to bring an air sickness bag.
You just need to follow the same steps you would with inserting or deleting at the top, but instead of copying all of Queue 1 to Queue 2, you'll only copy as many as you need to get the right element to the front.
Say you wanted to insert 5 into this queue in order, meaning that you'd want to set it between 6 and 4.
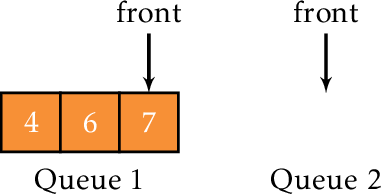
First step: copy over 7 and 6 to Queue 2.
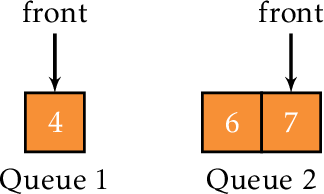
Then enqueue 5 into Queue 2, not Queue 1. If we enqueued it into Queue 1, that 5 would end up behind 4, when we want it coming ahead of 4. Plus, if we tried to put it all back together, we'd get 4, 5, 7, 6, which just isn't right. You have to enqueue it in Queue 2 to make it work.
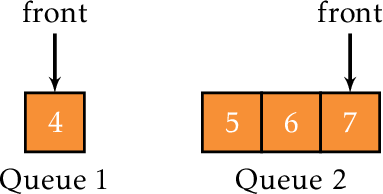
To finish things off, you'll have to move 4 into Queue 2. Now it has the new element and everything's in order. That's the dream.
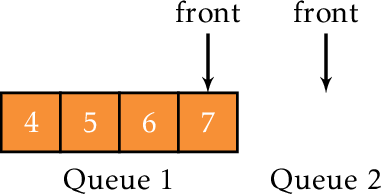
TL;DR: It's much easier to delete an element than it is to insert it. But whether you're inserting or deleting, if you're working against the way the data structure wants to work, be ready for that data structure to put up a fight.