Programming: Logic
Programming: Logic
Let's say you're going to write a program that simulates a game of chess. First things first: you need to set up a chessboard.
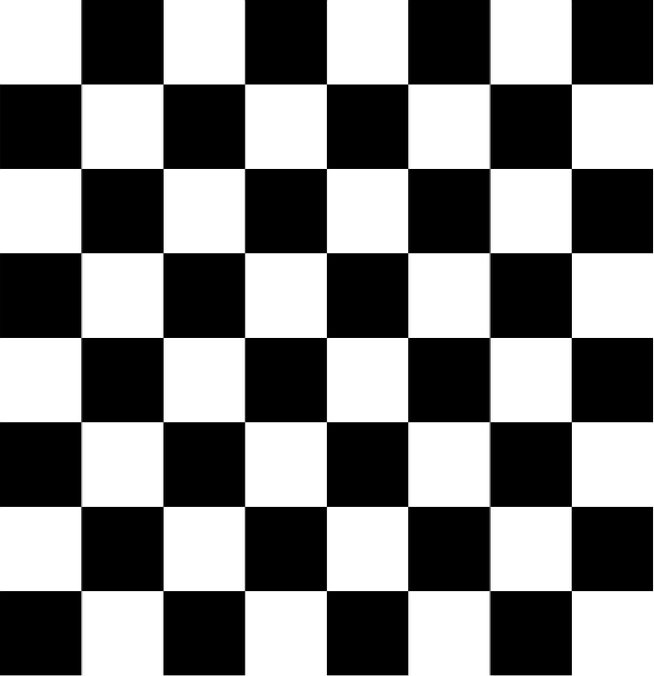
You need to come up with a way to tell the computer how to display the board, so you decide to use numbers. That's all the computer really knows after all. You decide to represent the black value as 0 and the white value as 1.
That works great until someone else looks at your code, assumes those numbers mean something else—like maybe which pieces have been taken by each side—and decides to add the numbers together. Adding together black and white? What does that even mean?
Boom. The program implodes.
That's why we need something more explicit than just plain numbers: we need True or False values, also known as booleans. If we set one to true and the other to false, there's much less room for confusion.
Try adding together our chessboard now, fellow coder!
While we understand boolean as true or false, the computer actually knows it as 1 or 0. Bear with us, here. Under the hood, a 0 is the absence of an electrical signal while a 1 is the presence of an electrical signal. That's the whole reason we use binary in computer science.
But that's not all we need when we talk about booleans: there's an entire language to build complex true or false statements. We won't go into the nitty-gritty of logic here, but in programming, there are some fundamental parts to Boolean logic.
Logical And
Logical and, usually represented with some ampersands (&), takes two boolean values, or two expressions that evaluate to boolean values, and tells you whether both values/expressions evaluate to "true."
Logical Or
Logical or, usually symbolized with some pipes (||), takes two operands—two boolean values or two expressions that evaluate to boolean values—and returns true if at least one operand is true. Both can be true, but we only need one value to be true for or to return true.
Logical Not
Not, usually symbolized with an explanation point (!), turns true booleans false and false booleans true. It can even take entire expressions and turn the result into its opposite.
Turning Numbers into Logic
When we're talking about logic, we don't always deal with booleans. That's right: numbers—and even characters—can be converted into true or false.
The following table is filled with relational operators, or operators that compare one value to another.
Sign | Meaning |
== | Equals |
!= | Is not equal to |
>= | Is greater than or equal to |
<= | Is less than or equal to |
> | Is greater than |
< | Is less than |
These operators only really work on primitives, since most programming languages compare the values of the variables. If the variables point to items in the RAM—regardless of whether or not the items are exactly the same—the operators compare the memory address, not the contents of the items.
The minute we start comparing primitives, though, we're creating booleans that tell us whether the comparison is true or not.