Foundations of Programming—Semester A
Changing your major to code.
- Course Length: 18 weeks
- Course Type: Basic
- Category:
- Technology and Computer Science
- High School
Schools and Districts: We offer customized programs that won't break the bank. Get a quote.

"The world today is more connected by technology than ever before."
Yawn.
Admittedly, this obvious sentiment might sound like something someone with a deep, booming voice would say at the beginning of an IBM commercial and…it probably is. That doesn't stop it from being true. The world's filled to the brim with computers. Why not learn how to use them?
We aren't talking about opening Microsoft Word or figuring out how to connect to a Wi-Fi printer (although both of those are very helpful—and money-making—skills to have). No, we're talking about delving into what's underneath all those shiny applications to understand how they were made.
In this yearlong course, we'll give you all the basics you need to build your own programs, using Java to cover important concepts like
- variables, objects, and arrays to store data.
- logic and conditions to make sure your code does exactly what you ask it to.
- loops to make the code repeat itself so that you don't have to.
- how to get fancy graphics showing up on the screen from code alone.
- what real-life computer product development feels like.
(Just imagine we said all that in that booming, IBM voice. Everything sounds better that way.)
This course is only the beginning to your computer science career. With it, you'll have all the tools you need to make interesting, complex projects. And, of course, games. Lots and lots of games. Plus, this course is aligned to the following computer science standards:
The point? Foundations of Programming is an invaluable entry point to all things comp sci. Whether or not you use it to make more fancy IBM commercials is up to you.
Technology Requirements
Make sure you've got a computer and an ability to download some Java-running tools. And...that's it.
Unit Breakdown
1 Foundations of Programming—Semester A - The Fundamentals of Coding
Before you can control the computer, you'll need to know a little about the computer. If you know how it works, you can figure out why something isn't working in the future. That's the theory, anyway. Get all the background deets here.
2 Foundations of Programming—Semester A - Running the Numbers with Variables
So much in life is variable, like how the cafeteria was had plenty of sporks yesterday but now only has forks and spoons. Computers need to deal with variables too…except they don't usually get excited and yell, "Viva la spork-olución!" about it. Here's the unit where you'll learn all about saving and storing basic values.
3 Foundations of Programming—Semester A - Taking Control of Your Code
Computers are literal and controlling, and that's a good thing. You can make your code so much more flexible when you guide the computer through complex decision-making processes using a handy little thing we like to call, "logic." Boolean logic, if we're getting technical.
4 Foundations of Programming—Semester A - Objectively Speaking
TBH, up until this unit, you could've been programming in any old language. In this unit, though, we'll start talking about something Java holds near and dear to its binary heart: objects. They're great data structures and Java was basically built entirely for them, so play nice.
5 Foundations of Programming—Semester A - Even More Control
We mentioned the computer was controlling, right? Because in this unit, you'll get even more tools for telling the computer exactly what to do, down to the smallest getter method. And you'll even become a super cryptographer while you're at it. Maybe.
6 Foundations of Programming—Semester A - Drawing by the Numbers
Sometimes, you've got to get a little graphic to get things done. In this unit, you'll learn all about Java's graphics package and how to make images show up on the screen—instead of just ASCII art. Not that there's anything wrong with ASCII art, but…
7 Foundations of Programming—Semester A - Life is Full of Lists
Life isn't all primitives and objects. Sometimes you need to make lists of those primitives and objects. With this data structure, you'll be able to take on way more data in your programming. Just make sure to mention Shmoop when you accept your Turing award for all the awesome arrays you've made.
8 Foundations of Programming—Semester A - Feel the UML
We're setting you loose on your own project in this unit, giving you control on the requirements, planning, and implementation of your very own game of Tic-Tac-Toe. Who knows? You could even turn the characters from Xs and Os to Is and ls if you want to. (Except…you probably wouldn't want to do that if you want people to actually play your game. We're just saying.)
Loading
Loading
Sample Lesson - Introduction
Lesson 1.05: The Language of Programs
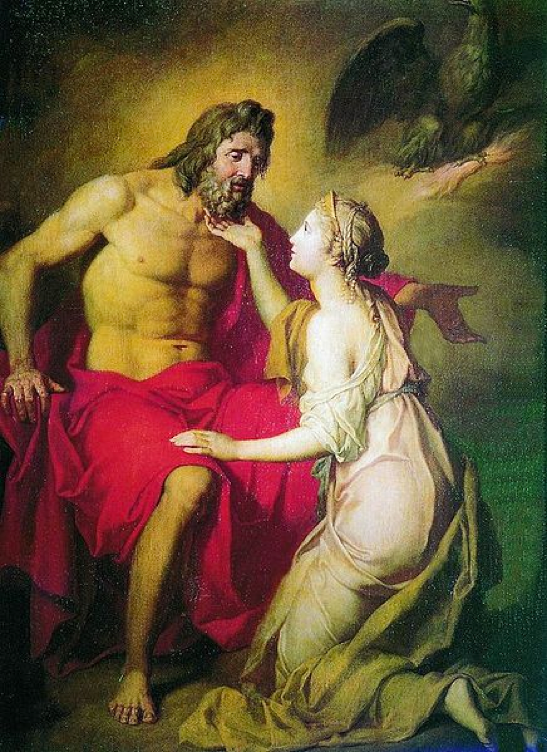
Rubbing the computer's chin probably doesn't help either. (Source)
When you think of computers, we're willing to bet your mind jumps to the words:
- Powerful
- Smart
- Magical
- Sinister
We'll ignore the fact that you just described your average Greek god. Instead, let's focus on dispelling one major assumption: that computers are smart. Computers are the opposite of smart. They can only do what they're told; they can't even figure out what to do if the information isn't spoon-fed into their input devices, step by excruciating step. In fact, the only thing they really get is circuits. If the circuit's on, that means one thing. If it's off, that's another thing (the opposite thing, by some estimates).
The problem? Humans aren't too good at thinking entirely in on and off switches. Sure, they can abstract away from them by representing on as 1 and off as 0, but talking in zeros and ones all day doesn't sound too fun, either.
They're mere mortals, after all.
In case you were wondering, the solution isn't to say that computers work in mysterious ways, set up a shrine, and burn incense. We've tried it; the computer didn't do anything. Instead, we're going to rely on the work of past computer scientists who made programs that could translate between raw electricity and human language. Programmers like Grace Hopper, who came up with the idea to teach computers English-like words, made it so that we can actually talk to computers.
Let's talk about the different languages we can work with today—without any frankincense or animal sacrifices.
Sample Lesson - Reading
Reading 1.1.05: The Robotic Tower of Babel
Way, way back in the day (also known as Lesson 3), we talked about Grace Hopper and her invention of the compiler: a piece of software that translated English keywords into specific computer commands. Most modern languages are built on this principle: ask the programmer to churn out English-like commands and make the computer translate it back to binary. (There are also programming languages that take commands in different human languages, but we'll ignore them for now.)
Unless the programming language is literally written in binary (which is a way to abstract away from circuits being on or off), in fact, it needs some sort of translator. There are two types to choose from: compilers and interpreters.
A compiler takes the code after it's written and converts it into computer language all in one go. In comparison, an interpreter is a little lazier; it doesn't convert the code into computer language until you actually run the program. Then it converts to machine code, line-by-line. That might sound like a small difference, but it's surprisingly large.
Either way, the history of programming languages is the history of using layers of code to abstract away from raw binary into instructions people can read. Let's take a minute to appreciate all the different types of computer languages there are before we zero in on Java forever.
Or at least for the rest of the course.
Category One: The Machine Languages
Most programmers you find on the street probably aren't going to call machine language or machine code an actual programming language, but when you get right down to it, that's all a computer actually understands. Machine language is a set of coded instructions that each different type of computer processor understands.
Computer language is actually just the transistors in the memory, each of which can either be on or off—0 or 1. Give a computer an English command and it'll sit there quietly. Send it something like the following piece of binary, and it'll have something to work with.
0100011111110101
The computer can go through and read which transistors are off, which ones are on, and figure out what to do based on whatever command was just given right there. (Unless the command was to sit there blankly. Then you're back at square one.)
Every processer has its own, unique way of understanding combinations of 0s and 1s. To make things even more complicated, even different types of hardware with the same processor could need different kinds of machine code. Programming on a computer that only takes machine code means knowing everything about how they were built.
And every different computer model has a different way of coding instructions. Early programmers had tons of fun writing and rewriting the same code to work on different computer architectures. So much fun, in fact, that many people compare trying to understand a program written in machine language with trying to understand DNA looking at it atom by atom.
As programing became more of a thing, people got tired of trying to use machine language. Fast. They started to develop easier and better ways to deliver answers. If you're one of those do-it-by-hand types, though, it's still possible to write programs directly in the numerical machine code of your system. Just make sure to buy a box of stress balls before you do.
Don't forget: everything you do on your computer—and we mean everything—eventually simplifies down to a series of zeros and ones when it hits the hardware of the machine.
Everything.
Category Two: Assembly Languages
Assembly language might be a small step up from machine language, but when it was new, it was a giant leap in allowing people to get work done with computers. Remember all the different languages written in pure binary? Yeah, so do early programmers' nightmares.
Your average assembly language uses a few English-style words that map directly to the machine language binary instruction. This language can get away with letting programmers use a little English by using a program called an assembler, which translates between the assembly language and the machine language for the computer.
This didn't save that much time, seeing as each instruction in assembly had a one-to-one relationship with an instruction in machine language, but it did save early programmers' sanity by keeping them away from all unique combinations of zeros and ones that ran a different operation. For example, instead of typing in 100000
and 100001
, now they could type ADD
and SUBTRACT
. We'll let you guess which commands were easier to memorize.
Since every computer has its own flavor of binary instructions, though, each one needs its own special assembly language. Just like with machine language, programming in an assembly language meant learning each assembly language for individual computers.
Category Three: High-Level Languages
Assembly language, while better than machine language, still had its own issues. To add two numbers and store the result needed several instructions in assembly. A programmer had to get the two numbers from memory (two instructions on their own), add them (the third instruction) and then store the result (that's the fourth instruction just for some basic addition). Programmers knew they could do better if they used more English and less machine code.
The idea was to create a language that the user wrote in English, which would then translate into assembly, which would then be translated into machine language. Once in machine language, the computer could run the commands and give some outputs.
The best part? If a programming language was abstracted away from assembly language, it didn't even need to know how the computer was built and what systems it used. Instead, it just needed a piece of software that could translate the language into whatever assembly language (and then machine language) the computer had. To translate from high-level language to low-level language (which is another way to call an assembly language), the language needs a translator in the form of either a compiler or an interpreter.
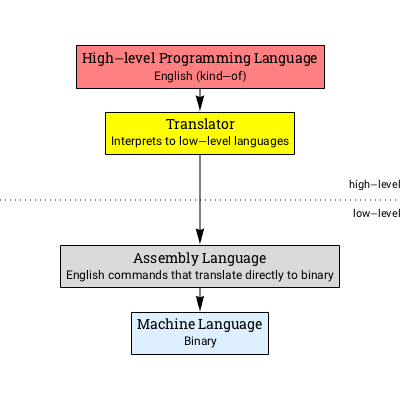
Now the only people who needed to know a computer's individual architecture were the ones who actually wrote the compiler or interpreter. Everyone else could learn the language that used said compiler or interpreter. Sounds much easier, if you ask us.
Interpreted languages are lazy: they wait to translate each line of the program into a lower level language when it runs. As a result, programs end up running much faster, but sometimes crash in the middle of running a program if there's an error.
Compiled languages, on the other hand, tend to be go-getters. They make programmers translate the entire program into a lower level language before they can be run. If you misspell something, the compiler's going to find it right away and throw an error without making you run the entire program to find it. Still, compiling a program takes an awful lot longer to set up and run, but once they're compiled, all the most basic errors will have already been found and fixed.
With high-level languages also came a more diverse set of specialties a program could have. When the first three high-level programming languages appeared in the 1950's, they were each built for a different purpose.
- FORTRAN, which stood for "FORmula TRANslation," was built with scientific calculations in mind.
- COBOL, or "COmmon Business Oriented Language," was meant for business and finance organizations. Bet you didn't see that one coming.
- LISP, also known as "LISt Processing language," was designed to program artificial intelligence applications.
All three are still in use today, but even more have cropped up in the past 60 years, too. Again, all with a specific purpose in mind, BASIC, ALGOL, Pascal, Ada, Prolog, Logo, Scheme, Smalltalk, Simula-8088, C, C++, Python, Java, Matlab, Perl, Lua, PHP, and Ruby are fairly commonplace nowadays.
And they're all excellent at keeping people from writing too much binary. You can thank their creators later.
Java, in Particular
Believe it or not, Java falls into the category of a high-level programming language. In fact, we probably wouldn't ask you to work with it for the rest of the course if it wasn't. Life's just too short to code in binary, you know? You'll learn more about Java soon, but for now just enjoy the fact that you won't even need to learn binary to use it.
You still will learn binary, though; you just won't have to use it every day. Whew.
Sample Lesson - Activity
Activity 1.05a: Lingua Brochura
Brochures are great. They give important information, like
- how to eat healthy.
- what a company does (or at least, what they want you to think they do).
- what smiling people look like without that pesky watermark.
Get ready to research a programming language that caught your attention, because you're about to make your very own brochure filled with impressively happy people and how they use a particular programming language.
Emphasis on the programming language bit.
Step One
Pick a high-level programming language, any programming language. Our recommendations are any of the following.
- Ada
- BASIC
- C
- JavaScript
- Matlab
- Prolog
- Python
You could pick a different language, but we'd recommend staying away from Java. You've got the rest of the year to deal with Java; take today to learn something different first.
Take some time and get to know your language. You should know the basics like who created it when (obvi), but also things like
- what it was originally created to do.
- how it's used now and why someone would choose it as a programming language.
- what kind of translator it uses and how it converts from English to binary.
- what its strengths are.
- what its weaknesses are.
We've given you a starting point for each language as a freebie (you're welcome), but you'll need to find two other pages that can tell a little more about your language. Make sure they're from .ORGs, .EDUs, or major publications. Otherwise, you won't be sure that the source is trustworthy, which is never fun.
By the time you're done, you should have enough notes to write a five to seven sentence paragraph on each topic. About three to five bullet points should be enough for that.
Once you've figured out all those details, you'll be ready for the next step.
Step Two
Open up your favorite brochure maker, like Microsoft Publisher, Adobe InDesign, or Canva if you're looking for something…free. Then set up the panels so that
- the cover has the name of your language, as well as your name.
- the back cover has the title "Works Cited" and the sources you found in the last step.
- a panel has a "History and Where It's Used Today" title.
- a panel has a "How it Translates from Human to Computer" title.
- a panel has a "Strengths" title.
- a panel has a "Weaknesses" title.
Step Three
Turn your bullet points into five to seven sentence paragraphs. They should include intro and conclusion sentences, as well as all the language-specific details you found in your research. For example, if Shmoop was taking some bullet points we wrote on the strengths and weaknesses of assembly languages:
- Originally created to abstract away from machine code
- Allowed programmers to use human language when communicating with computers
- Changed depending on the computer architecture and was limiting in power
- Still very hard to read
We'd write:
Assembly languages, which are often called a low-level programming languages, helped programmers start abstracting away from pure machine code. Instead of making programmers learn the different binary commands for instructions like adding and subtracting, assembly languages let them write the same basic commands in English, which would then be converted into binary by the computer. Because every command had one matching binary command in machine language, the commands changed with every new computer design, making programming limiting. It was also difficult for people to read.
We actually had to combine two sections because we were generalizing about all assembly languages. Don't do that. Make sure each section says something a little different about the programming language, if you know what we mean. You should still be writing in complete, grammatically correct, and typo-free sentences, though.
Once you've completed your paragraphs, proofread 'em and then add them to their panels in the brochure.
Step Four
Add some interesting and appropriate images to your brochure. You can totally add a theme to your brochure, too, but it isn't required. You should have a cool, related image in every panel when you're done. For example, Shmoop might use a picture of IBM 650 in our history section because it was one of the first computers that could be programmed using an assembly language.
Don't forget to include the URL of the site you took the image from and give a little caption to explain how that image relates.
When you've made your flawless brochure about your programming language, submit it below, and consider yourself a master of that language. Aside from knowing how to actually program in it, that is.
Creative Visual Presentation Rubric - 25 Points
Sample Lesson - Activity
- Course Length: 18 weeks
- Course Type: Basic
- Category:
- Technology and Computer Science
- High School
Schools and Districts: We offer customized programs that won't break the bank. Get a quote.
