Lists: Inserting and Deleting from Stacks
Lists: Inserting and Deleting from Stacks
Additions
If you need to put clean dishes away in your cupboard, you probably put them at the top of the stack. Try putting them in the middle or the bottom and you risk destroying all your plates.
Ten out of ten dish-stackers agree that the top is the easiest place to add new dishes. After that, it's probably easiest to add to the bottom (assuming you're better at balancing stacks fine china than we are), with the middle being the hardest to get at.
It's the same deal with stacks in computer science. Since elements are always added and removed from the top of the stack, the top is the easiest place to push to or pop from. If you want to add something to the bottom of the stack, you might want to change your data structure so that you're working with a queue instead. We're just throwing that out there.
Say you only need to do this once in a million times, though. If the stack happens to be empty, adding to the bottom would be fine, since the top and bottom would be the same place.
For non-empty stacks, it's difficult, but not the most impossible thing you could do in CS. (Did we mention this is a sign you're using the wrong data structure, though? Because it is.)
Here's a stack.
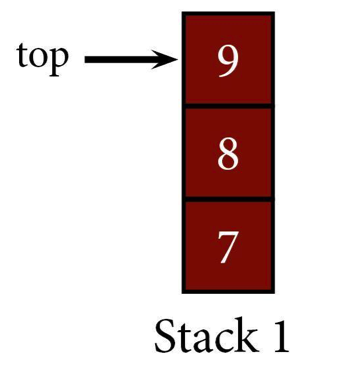
To insert 6 at the bottom of this stack, you'll need to create a separate stack and pop 9, 8, and 7, onto that second stack. They should be in reverse order, which isn't a big deal because you'll just be popping them back on to the original stack in a couple of seconds.
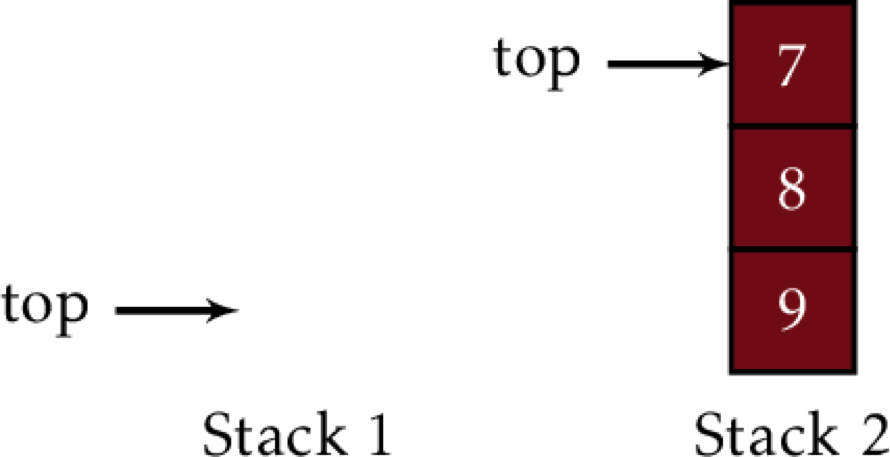
Then push 6 onto Stack 1.
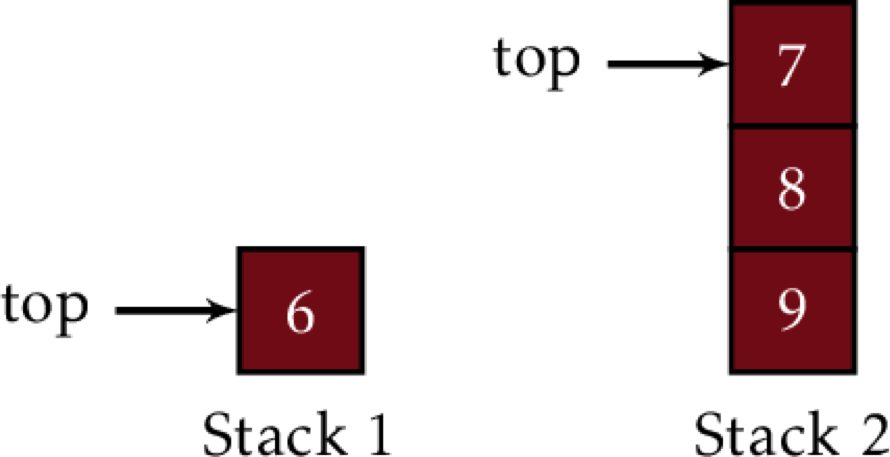
Now that you have 6 at the top/bottom, just pop off all the values in Stack 2 and push them back on to Stack 1. Because of how stacks pop from the top, the new Stack 1 will have the same order as before…plus the new element at the bottom.
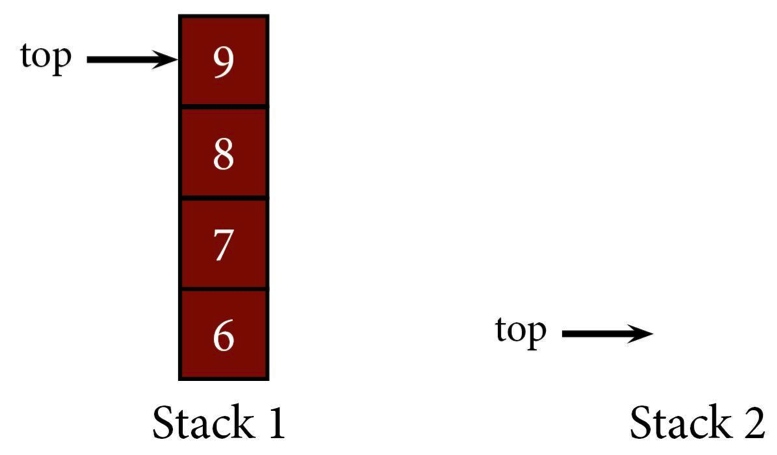
It took one operation (one push) to add to the top of that stack, but it took 13 whole operations (pushing and popping every element except 6 twice) to add to the bottom of the stack. And any time you add an element to the middle, you're doing about the same amount of work.
If you want to add elements to any place besides the top a bunch of times, the stack data structure isn't your answer. We're just sayin'.
Deletions
Say you want to delete something from the bottom instead. Let's work through deleting 2 from this list.
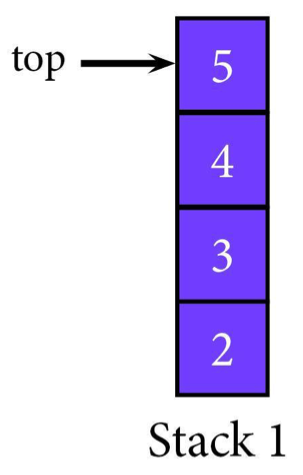
To delete it, you need to reach the bottom element, meaning you need to move everything to—wait for it—a second stack. Just like before, you'll start by popping off (and then pushing on) all of Stack 1 (except for 2, of course) to Stack 2.
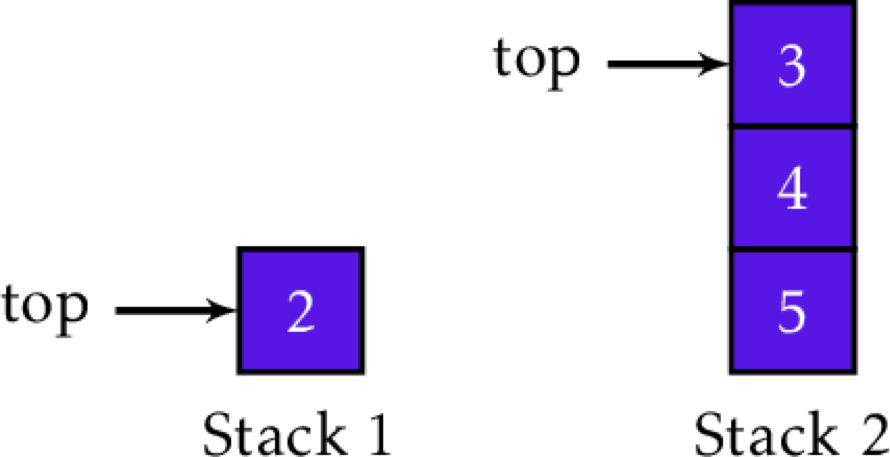
Then pop 2. If things need to stay in order, you can pop and push everything back to Stack 1. Otherwise, you're done.
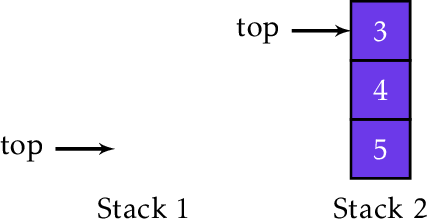
Deleting from the middle of the stack would be about as bad—even if you don't have to move everything twice.
Yup, you really shouldn't be using stacks if you constantly need to insert or delete elements from anywhere besides the top of the list.